In this article i will illustrate how to create a Sample web service and consume it.
Creating Simple Calculator Web Service
Create a new website and create a folder named “Web Service”.
Now select Add Item and Select “Web Service” from the templates and name it as “CreateSimpleCalculatorWebService”.
It Creates “CreateSimpleCalculatorWebService.asmx” in your folder and “CreateSimpleCalculatorWebService.cs” will be placed in “App_Code” folder.
Now if you see , by default “HelloWorld” web method definition will be there in the cs file.
[WebMethod] public string HelloWorld() { return "Hello World"; } |
[webMethod] , its represents attribute.
To make a function accessible or invoked remotely we need to place this [webMethod] with the function definition. And the function should take “public” access specifier only. if “Private” or “protected” is used it could not be accessed out side the class.
so we will write a function (WebMethod) which adds two numbers and returns the result as follows.
[WebMethod] public int addNums(int a, int b) { return a + b; } |
now execute this and you can see the following output.
To test the methods in your web service click on the hyperlink with that function name. Suppose when you click on the addnums hyperlink , it prompts for the user input and invokes the method on click of Invoke button.
Output window after entering the required input and clicking on invoke button.
Consume Web Service:
We have learnt to create a web service . Now we will see how to consume this in our project or website.
Right click the same folder “WebService” and Select “Add Item”. Select “WebForm” and name it “ConsumeWebService.aspx”.
Right click the solution and select “Add Web Reference” .
Next

Now Click on the link “Web Services in this solution” . It shows all the web services in your solution. As we have created “ CreateSimpleCalculatorWebService” web service , it will be shown in the list.
or
Copy paste the link of the web service that we have created and click “Go” . ( execute “CreateSimpleCalculatorWebService.asmx” and copy that link from the browser)
Now select the web service .It displays all the methods in the web service that are exposed. Give a any reference name to web service and click ok.and give a any reference name (say here I gave “SimpleCalculator”) and click on “Add Reference”
Now in “ConsumeWebService.aspx” add two text boxes to input two numbers and a “Add” button on click of which “addnum” web method is invoked and the result is displayed in result textbox.
To work with web services import this namespace “System.Web.Services;”
Aspx Code:
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="ConsumeWebService.aspx.cs" Inherits="Web_Service_ConsumeWebService" %> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> </head> <body> <form id="form1" runat="server"> <div> <table> <tr> <td> Enter a:<asp:TextBox ID="txta" runat="server"></asp:TextBox><br /> </td> </tr> <tr> <td> Enter b:<asp:TextBox ID="txtb" runat="server"></asp:TextBox><br /> </td> </tr> <tr> <td> <asp:Button ID="btnAdd" runat="server" Text="Add" OnClick="btnAdd_Click" /> </td> </tr> <tr> <td> Result:<asp:TextBox ID="txtResult" runat="server" Enabled="false"></asp:TextBox> </td> </tr> </table> </div> </form> </body> </html> |
create an object for the web service class using reference name and invoke the required web method.
SimpleCalculator.CreateSimpleCalculatorWebService obj = new SimpleCalculator.CreateSimpleCalculatorWebService();
|
Code Behind :
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.Services; public partial class Web_Service_ConsumeWebService : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { } protected void btnAdd_Click(object sender, EventArgs e) { SimpleCalculator.CreateSimpleCalculatorWebService obj = new SimpleCalculator.CreateSimpleCalculatorWebService(); txtResult.Text = Convert.ToString(obj.addNums(Int32.Parse(txta.Text) , Int32.Parse(txtb.Text))); } } |
Output:
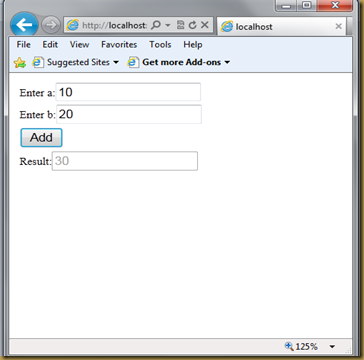